- DarkLight
Adobe Commerce 2: Checkout Module Implementation Example
- DarkLight
The Checkout module is a highly-customizable way to let customers redeem their points at checkout. This article provides an example of how to implement a Checkout Module on the Adobe Commerce 2 checkout page.
Implementing a Checkout module
In this example, we will be creating two directories - CustomCheckout and CustomCheckoutModule.
You can choose any name you would like for these directories, but make sure to adjust the code snippets to reflect the names you chose.
After connecting through SSH to your Adobe Commerce 2 machine, you can follow these steps to implement the Checkout Module:
- Create a new directory under /app/code called CustomCheckout
- In the CustomCheckout directory, create another directory called CustomCheckoutModule, and in it, add the following files and directories:
a. registration.php
<?php
use Magento\Framework\Component\ComponentRegistrar;
ComponentRegistrar::register(
ComponentRegistrar::MODULE,
'CustomCheckout_CustomCheckoutModule',
__DIR__
);
b. etc/module.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="CustomCheckout_CustomCheckoutModule" />
</config>
c. view/frontend
In the frontend dir, create:
i. layout/checkout_index_index.xml
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceBlock name="checkout.root">
<arguments>
<argument name="jsLayout" xsi:type="array">
<item name="components" xsi:type="array">
<item name="checkout" xsi:type="array">
<item name="children" xsi:type="array">
<item name="sidebar" xsi:type="array">
<item name="children" xsi:type="array">
<item name="summary" xsi:type="array">
<item name="children" xsi:type="array">
<item name="itemsBefore" xsi:type="array">
<item name="children" xsi:type="array">
<item name="testcustom" xsi:type="array">
<item name="component" xsi:type="string">CustomCheckout_CustomCheckoutModule/js/view/summary/custom</item>
</item>
</item>
</item>
</item>
</item>
</item>
</item>
</item>
</item>
</item>
</argument>
</arguments>
</referenceBlock>
</body>
</page>
This example represents a tree that injects the checkout module into the order summary section.
When implementing, make sure to adjust the XML tree according to your checkout page and the location you would like the module to be injected into.
ii. web/
1. template/summary/custom.html
<div class="yotpo-widget-instance" data-yotpo-instance-id="#INSTANCE-ID#" data-bind="afterRender: loadJsCustomAfterKoRender"></div>
The afterRender callback function is used to ensure that the JS is loaded after the module is injected into the page, making sure it exists when the JS is loaded
Please make sure to update the data-yotpo-instance-id with your Checkout Module instance ID.
To find your instance ID, please follow this guide.
2. js/view/summary/custom.js
define(
[
'uiComponent'
],
function (Component) {
'use strict';
return Component.extend({
defaults: {
template: 'CustomCheckout_CustomCheckoutModule/summary/custom'
},
loadJsCustomAfterKoRender: function() {
var script = document.createElement('script');
script.src = 'https://cdn-widgetsrepository.yotpo.com/v1/loader/X-GUID-X'
script.setAttribute('src_type', 'url')
document.head.appendChild(script)
}
});
}
);
Please make sure, when using this code snippet, to add the GUID to the end of the loader JS (line 13).
You can find your full loader JS path when publishing a module:
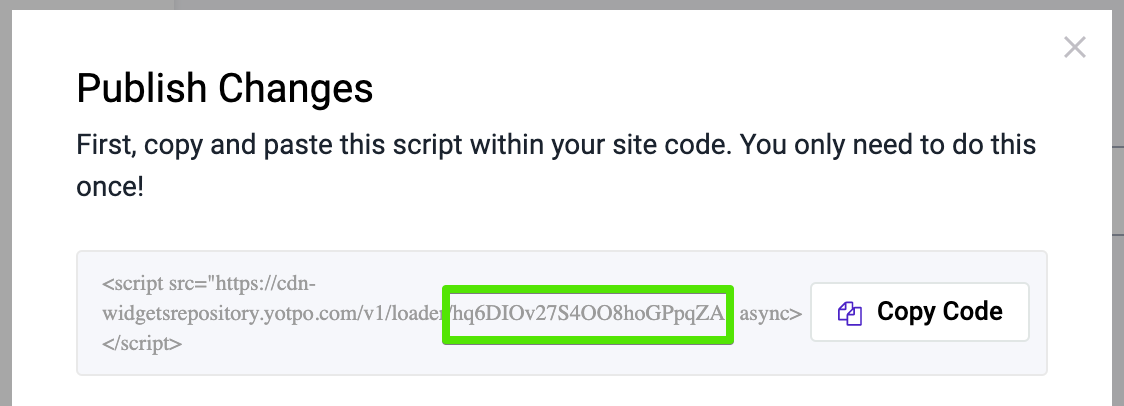
When copying the loader JS from the module, make sure not to add the async reference as it is important that this JS is loaded after the module was injected into the page.